Get your feet wet with Sun's tiny Java DB
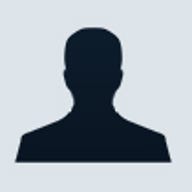
Java DB is a lightweight database management system (DBMS) that is written completely in Java. It only needs 2 MB of memory, and it makes it very easy to embed a fully functional database directly into your application. Java DB is also standards-based, supports SQL and the JDBC API, and easily integrates with J2EE. Beginning with the release of Java 6, Sun started packaging Java DB in the JDK.
The technology behind the DBMS
Apache Derby is the core technology of Java DB. Derby's database engine is a fully functioning relational embedded database engine. JDBC and SQL are the main programming APIs.
Another core component that supplements the Java DB is the Derby network server. The network server extends the reach of the Derby database engine by providing traditional client server functionality. The network server allows clients to connect over TCP/IP using the standard DRDA protocol; it also allows the Derby engine to support networked JDBC, ODBC/CLI, Perl, and PHP.
Derby has three useful utilities: ij, dblook, and sysinfo.
- ij is a tool that allows SQL scripts to execute against any JDBC database.
- dblook is a schema extraction tool for a Derby database.
- sysinfo is a utility that allows you to display version numbers and class path.
Multithreading concerns
When an application accesses the Derby database using the Embedded Derby JDBC driver, the Derby engine does not run in a separate process, and there are no separate database processes to start up and shut down. Instead, the Derby database engine runs inside the same Java Virtual Machine (JVM) as the application; the Derby actually becomes part of the application just like any other jar file that the application uses.
Derby supports multiple connections to a given database. An example of an embedded application that manages concurrent users is a Tomcat or Geronimo application server that embeds Derby. Any number of users can execute Web applications that access a database through that Web server. Only one JVM may boot ("open") that database, so multiple applications running in different JVMs cannot access the same database.
Get started with Java DB
In order to start using the DBMS, you need to download Java DB from the Sun Developer Network. The binary distribution provides everything you need to begin working with embedded database applications.
After you download the binary distribution, the Java DB directory structure contains these subdirectories: demo, frameworks, javadoc, docs, and lib.
- demo has two demonstration programs. One example shows how to create a trivial embedded application, and the other example shows how to use Java DB in a client-server environment.
- frameworks contains utilities for setting up environment variables and for creating and working with databases. (I do not use this subdirectory in the demo I outline in this article because our application will be entirely self-contained. No outside utilities will be used for the Address Book application.)
- javadoc contains API documentation. This subdirectory is particularly useful if you configure your IDE to point to it as the Java DB API Javadoc.
- docs contains documentation about the Java DB product setup, administrator, and reference guides.
- lib contains the Java DB libraries packaged as JAR files.
(Read the Java DB documentation to find out about the various libraries.)
For an embedded database application, I will use only the derby.jar library file; to install Java DB for development, it only requires that you make the derby.jar file part of your application classpath.
After you install the binary distribution and include derby.jar into your classpath (if you've already installed Java 6, simply include the derby.jar into your classpath), you can start using Java DB just like you would any other JDBC-compliant database.
Driver management
You can start using the DBMS by loading the JDBC technology driver. (Java DB's drivers come with the derby.jar file, so you don't need to download anything else.) To load the driver, reference it using the Class.forName method. The embedded driver name is org.apache.derby.jdbc.EmbeddedDriver, and you can load it as you would other JDBC drivers:
Class.forName("org.apache.derby.jdbc.EmbeddedDriver");
When you want to retrieve a connection from the driver manager, you simply provide a URL string that identifies the database and a set of properties that influence the connection's interaction with the database. A very common use of properties is to associate a user name and password with a connection. The dbName portion of the URL identifies a specific database. A database can be in one of many locations, including: the current working directory, a JAR file, a specific Java DB database home directory, an absolute location on your file system, or on the classpath.
The easiest way to manage your database location in an embedded environment is to set the derby.system.home system property; this property tells Java DB the default home location of all databases. The optional propertyList is a set of properties that you can pass to the database system. You can pass properties into the Java DB system either on the URL or as a separate Properties object. If properties are part of the URL, a semicolon (;) should precede each property. Here are the most common properties:
create=true databaseName=nameOfDatabase user=userName password=userPassword shutdown=true
Once the application specifies where all databases will exist, it can retrieve a database connection.
private void setDBSystemDir() { // Decide on the db system directory: <userhome>/.addressbook/ String userHomeDir = System.getProperty("user.home", "."); String systemDir = userHomeDir + "/.addressbook"; // Set the db system directory. System.setProperty("derby.system.home", systemDir); } Connection dbConnection = null; String strUrl = "jdbc:derby:DefaultAddressBook;user=dbuser;password=dbuserpwd"; try { dbConnection = DriverManager.getConnection(strUrl); } catch (SQLException sqle) { sqle.printStackTrace(); }
Have fun with this tiny database, which lives directly in your application.
Peter V. Mikhalenko is a Sun certified professional who works as a business and technical consultant for several top-tier investment banks.