Create an XML-based shopping cart in PHP
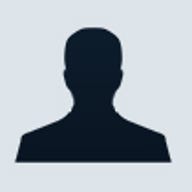
The reason for using XML to store and manage your shopping cart data is its portability, ease of use, and the growing support for XML/DOM in most scripting languages.
Also, the data can be serialized within the page and passed from page to page as hidden form data. Or, it can be passed back asynchronously to the Web server through Microsoft's XMLHTTP object or Mozilla'sXMLHttpRequest object, which is useful when you're binding XML data to form data elements.
The method
In its simplest form a shopping cart stores unique item
identifiers and a quantity value for each item. For example, here's how the XML may look:
<items>
<item><id/><qty/></item>
</items>
Each item in the items parent is an item record. The rest of the information is the minimal amount of information necessary to provide the functionality needed. Each item id represents a unique identifier in a catalog database. When the shopping cart detail is requested, the id is used to look up the detail information for each item in the database. The total price for the item is calculated using the quantity specified, qty.
Behind the scenes every page is a new connection so the shopping cart can be viewed as an object that must be instantiated with every page request.
So the XML is the serialized object with an items collection member. The XML is passed to the shopping cart constructor to create the shopping cart object with the items collection. Then, the shopping cart object can then contain a method for adding a new item through a member function called add_item. The add_item method simply adds a new item to the items collection member. Finally, an xml member function returns the serialized shopping cart.
Listing A contains the sample code. (Note: All the code in this article was tested and successfully run in PHP version 4.3.10 on Windows 2000 Advanced Server.)
The constructor of the Cart class creates an XML parser handle, which is used to specify the beginning and ending tag callback functions. These functions are called when a new XML tag opens or closes.
Using a couple of member variables, the id information is stored in the character data callback function cdata(). When a tag opens for id, the bIsID member variable is set to true. This enables the character data handler to set the cur_id member variable. When the qty tag opens, the bIsQty member variable is set to true, and the quantity specified by this tag is then updated in the appropriate items collection item during the character data callback. When the tags close, the appropriate member variables are set to false to avoid conflicts.
When all the XML is parsed, the items collection will contain a key/value pair where the key is the item id and the value is the quantity.
In order to use this class, you would require() it in any of your PHP pages that require the shopping cart information. The serialized data must then be passed in during the instance creation of the object. Then you would add or remove items or update the quantity for any item as needed.
Listing B features a simplified example using this class.
When you click the Add To Cart link on each list item, the itemId form data element value is set to the appropriate id passed in as the parameter of the addToCart() function.
The form is submitted and the data is updated. The updated quantity is displayed to the right of each list item. The serialized object data is stored in the cart form data element.
Another great thing about storing the shopping cart data in XML format is that when it comes time to create the View Shopping Cart functionality, you can simply run this through an XSL transformation. Any updates to data can be set just as it was in this simple example.
Phillip Perkins is a contractor with Ajilon Consulting. His experience ranges from machine control and client server to corporate intranet applications.