Create your first JSP page
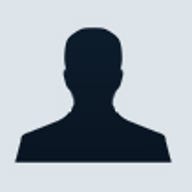
quote.jsp
First, create a quote.jsp page with the code
below and save it in the Web pages directory under the JSWDK
installation directory. Most of the page is standard HTML with JSP code
interspersed. Line 6 is a page directive that says to send any error to
the errorPage.jsp file. Lines 13 through 15 are a scriptlet that
basically says only show the table if the "symbol" parameter is
present. This if-block is closed on lines 32 through 34. Line 17
defines the JavaBean to use, and line 18 loads its symbol property from
the parameter. Lines 27 through 29 just display the bean properties.
Other than the if-block, there is really no Java coding involved.
<html>
<head>
<title>Stock Quotes</title>
</head>
<body>
<%@ page errorPage="errorPage.jsp" %>
<form action="quote.jsp"
method="GET"> <p>Enter Symbol: <input size="20" name="symbol"><input
type="submit" value="Submit"></p>
</form>
<%if (request.getParameter("symbol") != null) {
%>
<jsp:useBean id="quotes" scope="page" class="com.jguru.Quotes" />
<jsp:setProperty name="quotes" property="*" />
<table border="1">
<tr>
<th align="left">Symbol</th>
<th align="left">Name</th>
<th align="left">Price</th>
</tr>
<tr>
<td><jsp:getProperty name="quotes" property="symbol" /></td>
<td><jsp:getProperty name="quotes" property="name" /></td>
<td><jsp:getProperty name="quotes" property="price" /></td>
</tr>
</table>
<%
}
%>
</body>
</html>
errorPage.jsp
Next, save the following JSP source to the file
errorPage.jsp in the Web pages directory. The clue that this is an
error page is the first line, which sets the page directive isErrorPage
attribute to true. Whereas the prior page said where the error page is,
this page says it is the error page. The only other JSP-specific code
in the JSP file is the access to the implicit exception object. The
page just displays its value:
<%@ page isErrorPage="true" %>
<html>
<head>
<title>Error Page</title>
</head>
<body>
<h1>Our Error Page</h1></font>
<!-- Print Exception -->
We got ourselves an exception:
<%= exception %>
<a href="quote.jsp">Restart</a>
</body>
</html>
Quotes.java
The Quotes JavaBean uses a Yahoo resource to get
the stock pricing. Save the Quotes.java source (below) in the
quotes.java file in the classes\com\jguru directory under the JSWDK
installation directory. From there, compile it with the javac compiler
from the JSDK.
package com.jguru; import java.util.*; import java.net.*; import java.io.*; public class Quotes { String symbol; String name; String price; public void setSymbol(String symbol) { this.symbol = symbol; getSymbolValue(symbol); } public String getSymbol() { return symbol; } public String getName() { return name; } public String getPrice() { return price; } private void getSymbolValue(String symbol) { String urlString = "http://quote.yahoo.com/download/javasoft.beans?SYMBOLS=" + symbol + "&format=nl"; try { URL url = new URL(urlString); URLConnection con = url.openConnection(); InputStream is = con.getInputStream(); InputStreamReader isr = new InputStreamReader(is); BufferedReader br = new BufferedReader(isr); String line = br.readLine(); StringTokenizer tokenizer = new StringTokenizer(line,","); name = tokenizer.nextToken(); name = name.substring(1, name.length()-2); // remove quotes price = tokenizer.nextToken(); price = price.substring(1, price.length()-2); // remove quotes } catch (IOException exception) { System.err.println("IOException: " + exception); } } }
Once you've created the two JSP files, as well as created the JavaBean source file and compiled it, you can load up the quote.jsp file to see the results from http://localhost:8080/quote.jsp, assuming you haven't changed the JSWDK installation to use a different port. The page can definitely be prettied up, but it does what it needs to do functionally and is a good demonstration of JSP's capabilities.