Two ways to work more effectively with Java exceptions
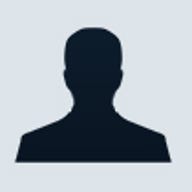
Nesting an exception
What should you do if you are catching an exception and want to throw a new one, but you still want the original exception's information to be available?
Try creating a NestedException class. This is easy to do, and the only major issues to deal with are providing enough constructors and overriding printStackTrace() so that it prints out data properly.
You should also consider wrapping a Throwable and not just an Exception class to create a more reusable component. Then, you can create a NestedRuntimeException variant that can wrap a Throwable but not need to be declared. Listing A presents a complete example.
Catching the right exceptions
Dealing with exceptions properly is a difficult balance to achieve, since they sometimes result in obscure program behaviors. However, following three simple rules will help you avoid the risks of poorly handled exceptions.
Rule #1: Always catch the type of exception being thrown and not a superclass. The snippets of code in Listing B show the wrong way and the right way to write this code.
To obey a common code convention, use the capital letters of the Exception class for the variable name, such as:
catch(FileNotFoundException fnfe)
and
catch(SQLException sqle)
Rule # 2: Never leave a catch block empty. Too often, try/catch blocks are written but nothing is done in the catch part of the block. Always print at least the stack trace. Or, if a Logging API is used, write the exception to the log.
Listing C shows the wrong way and the right way to handle this.
Rule # 3: Never throw an instance of the base Exception class. Developers should always create their own exceptions to throw.
An API that throws an exception is difficult to deal with. By declaring that a method throws java.lang.Exception, all the issues in Listing A are forced upon the API users, so they can't deal with exceptions professionally. By declaring subclasses of the Exception class for the API to throw, the API developer makes the user's life much easier.
Conclusion
The two techniques presented may be used to better or more appropriately handle exceptions. Nesting allows exceptions to be thrown inside another exception and catching the correct exception makes debugging easier.